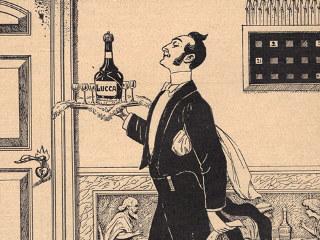
Even though there was somewhat of a delay since my last post in this series, it seems no-one else has really covered any of the advanced use cases of Drupal 8 in tutorials yet. So, here is the next installment in my series. I initially wanted to cover creating a new plugin type, but since that already requires creating a new servive, I thought I'd cover that smaller part first and then move on to plugin types in the next post.
I realize that now already a lot more people have started on their Drupal 8 modules, but perhaps this will make this series all the more useful.
Services in Drupal 8
First, a short overview of what a service even is. Basically it is a component (represented as a class) providing a certain, limited range of functionality. The database is a service, the entity manager (which is what you now use for loading entities) is a service, translation, configuration – everything handled by services. Getting the current user – also a service now, ridding us of the highly unclean global variable.
In general, a lot of what was previously a file in includes/
containing some functions with a common prefix is now a service (or split into multiple services).
The upsides of this is that the implementation and logic is cleanly bundled and properly encapsulated, that all these components can easily be switched out by contrib or later core updates, and that these systems can also be very well tested with unit tests. Even more, since services can be used with dependency injection, it also makes it much easier to test all other classes that use any of these services (if they can use dependency injection and do it properly).
(For reference, here is the official documentation on services.)
Dependency injection
This has been covered already in a lot of other blog posts, probably since it is both a rather central concept in Drupal 8, and a bit complicated when you first encounter it. However, before using it, I should still at least skim over the topic. Feel free to skip to the next heading if you feel you already know what dependency injection is and how it roughly works in Drupal 8.
Dependency injection is a programming technique where a class with external dependencies (e.g., a mechanism for translating) explicitly defines these dependencies (in some form) and makes the class which constructs it responsible for supplying those dependencies. That way, the class itself can be self-contained and doesn't need to know about where it can get those dependencies, or use any global functions or anything to achieve that.
Consider for example the following class:
<?php
class ExampleClass {
public function getDefinition() {
return array(
'label' => t('example class'),
'type' => 'foo',
);
}
}
?>
For translating the definition label, this explicitly uses the global t()
function. Now, what's bad about this I, hear you ask, it worked well enough in Drupal 7, right?
The problem is that it becomes almost impossible to properly unit-test that method without bootstrapping Drupal to the point where the t()
function becomes available and functional. It's also more or less impossible to switch out Drupal's translation mechanism without hacking core, since there is no way to redirect the call to t()
.
But if translation is done by a class with a defined interface (in other words, a service), it 's possible to do this much cleaner:
<?php
class ExampleClass {
public function __construct(TranslationServiceInterface $translation) {
$this->translation = $translation;
}
public function getDefinition() {
return array(
'label' => $this->translation->translate('example class'),
'type' => 'foo',
);
}
}
?>
Then our example class just has to make it easily possible for code that wants to instantiate it to know how to pass its dependencies to it. In Drupal, there are two ways to do this, depending on what you are creating:
- Services, which themselves use dependency injection to get their dependencies (as you will see in a minute) have a definition in a YAML file that exactly states which services need to be passed to the service's constructor.
- Almost anything else (I think) uses a static
create()
method which just receives a container of all available services and is then responsible for passing the correct ones to the constructor.
In either case, the idea is that subclasses/replacements of ExampleClass
can easily use other dependencies without any changes being necessary to code elsewhere instantiating the class.
Creating a custom service
So, when would you want to create your own service in a module? Generally, the .module
file should more or less only contain hook implementations now, any general helper functions for the module should live in classes (so they can be easily grouped by functionality, and the code can be lazy-loaded when needed). The decision to make that class into a service then depends on the following questions:
- Is there any possibility someone would want to swap out the implementation of the class?
- Do you want to unit-test the class?
- Relatedly, do you want dependency injection in the class?
I'm not completely sure myself about how to make these decisions, though. We're still thinking about what should and shouldn't be a service in the Search API, currently there is (apart from the ones for plugins) only one service there:
The "Server task manager" service
The "server tasks" system, which already existed in D7, basically just ensures that when any operations on a server (e.g., removing or adding an index, deleting items, …) fails for some reason (e.g., Solr is temporarily unreachable) it is regularly retried to always ensure a consistent server state. While in D7 the system consisted of just a few functions, in D8 it was decided to encapsulate the functionality in a dedicated service, the "Server task manager".
Defining an interface and a class for the service
The first thing you need, so the service can be properly swapped out later, is an interface specifying exactly what the service should be able to do. This completely depends on your use case for the service, nothing to keep in mind here (and also no special namespace or anything). In our case, for server tasks:
<?php
namespace Drupal\search_api\Task;
interface ServerTaskManagerInterface {
public function execute(ServerInterface $server = NULL);
public function add(ServerInterface $server, $type, IndexInterface $index = NULL, $data = NULL);
public function delete(array $ids = NULL, ServerInterface $server = NULL, $index = NULL);
}
?>
(Of course, proper PhpDocs are essential here, I just skipped them for brevity's sake.)
Then, just create a class implementing the interface. Again, namespace and everything else is completely up to you. In the Search API, we opted to put interface and class (they usually should be in the same namespace) into the namespace \Drupal\search_api\Task
. See here for their complete code.
For this post, the only relevant part of the class code is the constructor (the rest just implements the interface's methods):
<?php
class ServerTaskManager implements ServerTaskManagerInterface {
public function __construct(Connection $database, EntityManagerInterface $entity_manager) {
$this->database = $database;
$this->entity_manager = $entity_manager;
}
}
?>
As you can see, we require the database connection and the entity manager as dependencies, and just included them in the constructor. We then save them to properties to be able to use them later in the other methods.
Now we just need to tell Drupal about our service and its dependencies.
The services.yml
file
As mentioned earlier, services need a YAML definition to work, where they also specify their dependencies. For this, each module can have a MODULE.services.yml
file listing services it wants to publish.
In our case, search_api.services.yml
looks like this (with the plugin services removed):
services:
search_api.server_task_manager:
class: Drupal\search_api\Task\ServerTaskManager
arguments: ['@database', '@entity.manager']
As you see, it's pretty simple: we assign some ID for the service (search_api.server_task_manager
– properly namespaced by having the module name as the first part), specify which class the service uses by default (which, like the other definition keys, can then be altered by other modules) and specify the arguments for its constructor (i.e., its dependencies). database
and entity.manager
in this example are just IDs of other services defined elsewhere (in Drupal core's core.services.yml
, in this case).
There are more definition keys available here, and also more features that services support, but that's more or less the gist of it. Once you have its definition in the MODULE.services.yml
file, you are ready to use your new service.
Using a service
You already know one way of using a service: you can specify it as an argument for another service (or any other dependency injection-enabled component). But what if you want to use it in a hook, or any other place where dependency injection is not available (like entities, annoyingly)?
You simply do this:
<?php
/** @var \Drupal\search_api\Task\ServerTaskManagerInterface $server_task_manager */
$server_task_manager = \Drupal::service('search_api.server_task_manager');
$server_task_manager->execute();
?>
That's it, now all our code needing server tasks functionality benefits from dependency injection and all the other Drupal 8 service goodness.
Other posts in this series
- Part 1: Creating an entity type
- Part 2: Configuration and schema
- Part 4: Creating plugin types
- Part 5: Using plugin derivatives
Image credit: Didi
Comments
Since D8 Search API already
Since D8 Search API already kind of works I tihnk it would be good to move it form the sandbox into the official project page.
Already on it
Yes, you are right, and in fact this is exactly what we are currently doing: see #2231001: [meta] Search API 8.x path to its home repository.
We are just cleaning up the code (for which it is more practical when everyone can just commit) and then I'm going to move it back to its proper project. Should be there in a week or two, I'd guess.
Add new comment