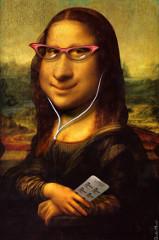
The greatest thing about all the refactoring in Drupal 8 is that, in general, a lot of those special Drupalisms used nowhere else were thrown out and replaced by sound design patterns, industry best practices and concepts that newcomers from other branches of programming will have an easy time of recognizing and using. While I can understand that this is an annoyance for some who have got used to the Drupalisms (and who haven't got a formal education in programming), as someone with a CS degree and a background in Java I was overjoyed at almost anything new I learned about Drupal 8, which, in my opinion, just made Drupal so much cleaner.
But, of course, this has already been discussed in a lot of other blog posts, podcasts, sessions, etc., by a lot of other people.
What I want to discuss today is one of the few instances where it seems this principle was violated and a new Drupalism, not known anywhere else (as far as I can tell, at least – if I'm mistaken I'd be grateful to be educated in the comments), introduced: plugin derivatives.
Probably some of you have already seen it there somewhere, especially if you were foolish enough to try to understand the new block system (if you succeeded, I salute you!), but I bet (or, hope) most of you had the same reaction as me: a very puzzled look and an involuntary “What the …?” In my case, this question was all the more pressing because I first stumbled upon plugin derivatives in my own module – Frédéric Hennequin had done a lot of the initial work of porting the module and since there was a place where they fit perfectly, he used them. Luckily, I came across this in Szeged where Bram Goffings was close by and could explain this to me slowly until it sank in. (Looking at the handbook documentation now, it actually looks quite good, but I remember that, back then, I had no idea what they were talking about.)
So, without (even) further ado, let me now share this arcane knowledge with you!
What, and why, are plugin derivatives?
The problem
Plugin derivatives, even though very Drupalistic (?), are actually a rather elegant solution for an interesting (and pressing) problem: dynamically defining plugins.
For example, take Search API's "datasource" plugins. These provide item types that can be indexed by the Search API, a further abstraction from the "entity" concept to be able to handle non-entities (or, indeed, even non-Drupal content). We of course want to provide an item type for each entity type, but we don't know beforehand which entity types there will be on a site – also, since entities can be accessed with a common API we can use the same code for all entity types and don't want a new class for each.
In Drupal 7, this was trivial to do:
<?php
/**
* Implements hook_search_api_item_type_info().
*/
function search_api_search_api_item_type_info() {
$types = array();
foreach (entity_get_property_info() as $type => $property_info) {
if ($info = entity_get_info($type)) {
$types[$type] = array(
'name' => $info['label'],
'datasource controller' => 'SearchApiEntityDataSourceController',
'entity_type' => $type,
);
}
}
return $types;
}
?>
Since plugin definition happens in a hook, we can just loop over all entity types, set the same controller class for each, and put an additional entity_type
key into the definition so the controller knows which entity type it should use.
Now, in Drupal 8, there's a problem: as discussed in the previous part of this series, plugins now generally use annotations on the plugin class for the definition. That, in turn, would mean that a single class can only represent a single plugin, and since you can't (or at least really, really shouldn't) dynamically define classes there's also not really any way to dynamically define plugins.
One possible workaround would be to just use the alter hook which comes with nearly any plugin type and dynamically add the desired plugins there – however, that's not really ideal as a general solution for the problem, especially since it also occurs in core in several places. (The clearest example here are probably menu blocks – for each menu, you want one block plugin defined.)
The solution
So, as you might have guessed, the solution to this problem was the introduction of the concept of derivatives. Basically, every time you define a new plugin of any type (as long as the manager inherits from DefaultPluginManager
you can add a deriver
key to its definition, referencing a class. This deriver class will then automatically be called when the plugin system looks for plugins of that type and allows the deriver to multiply the plugin's definition, adding or altering any definition keys as appropriate. It is, essentially, another layer of altering that is specific to one plugin, serves a specific purpose (i.e., multiplying that plugin's definition) and occurs before the general alter hook is invoked.
Hopefully, an example will make this clearer. Let's see how we used this system in the Search API to solve the above problem with datasources.
How to use derivatives
So, how do we define several datasource plugins with a single class? Once you understand how it works (or what it's supposed to do) it's thankfully pretty easy to do. We first create our plugin like normally (or, just copy it from Drupal 7 and fix class name and namespace), but add the deriver
key and internally assume that the plugin definition has an additional entity_type
key which will tell us which entity type this specific datasource plugin should work with.
So, we put the following into src/Plugin/SearchApi/Datasource/ContentEntityDatasource.php
:
<?php
namespace Drupal\search_api\Plugin\SearchApi\Datasource;
/**
* @SearchApiDatasource(
* id = "entity",
* deriver = "Drupal\search_api\Plugin\SearchApi\Datasource\ContentEntityDatasourceDeriver"
* )
*/
class ContentEntityDatasource extends DatasourcePluginBase {
public function loadMultiple(array $ids) {
// In the real code, this of course uses dependency injection, not a global function.
return entity_load_multiple($this->pluginDefinition['entity_type'], $ids);
}
// Plus a lot of other methods …
}
?>
Note that, even though we can skip even required keys in the definition (like label
here), we still have to set an id
. This is called the "plugin base ID" and will be used as a prefix to all IDs of the derivative plugin definitions, as we'll see in a bit.
The deriver
key is of course the main thing here. The namespace and name are arbitrary (the standard is to use the same namespace as the plugin itself, but append "Deriver" to the class name), the class just needs to implement the DeriverInterface
– nothing else is needed. There is also ContainerDeriverInterface
, a sub-interface for when you want dependency injection for creating the deriver, and an abstract base class, DeriverBase
, which isn't very useful though, since the interface only has two methods. Concretely, the two methods are: getDerivativeDefinitions()
, for getting all derivative definitions, and getDerivativeDefinition()
for getting a single one – the latter usually simply a two-liner using the former.
Therefore, this is what src/Plugin/SearchApi/Datasource/ContentEntityDatasourceDeriver.php
looks like:
<?php
namespace Drupal\search_api\Plugin\SearchApi\Datasource;
class ContentEntityDatasourceDeriver implements DeriverInterface {
public function getDerivativeDefinition($derivative_id, $base_plugin_definition) {
$derivatives = $this->getDerivativeDefinitions($base_plugin_definition);
return isset($derivatives[$derivative_id]) ? $derivatives[$derivative_id] : NULL;
}
public function getDerivativeDefinitions($base_plugin_definition) {
$base_plugin_id = $base_plugin_definition['id'];
$plugin_derivatives = array();
foreach (\Drupal::entityManager()->getDefinitions() as $entity_type_id => $entity_type_definition) {
if ($entity_type_definition instanceof ContentEntityType) {
$label = $entity_type_definition->getLabel();
$plugin_derivatives[$entity_type_id] = array(
'id' => $base_plugin_id . PluginBase::DERIVATIVE_SEPARATOR . $entity_type_id,
'label' => $label,
'description' => $this->t('Provides %entity_type entities for indexing and searching.', array('%entity_type' => $label)),
'entity_type' => $entity_type_id,
) + $base_plugin_definition;
}
}
return $plugin_derivatives;
}
}
?>
As you see, getDerivativeDefinitions()
just returns an array with derivative plugin definitions – keyed by what's called their "derivative ID" and their id
key set to a combination of base ID and derivative ID, separated by PluginBase::DERIVATIVE_SEPARATOR
(which is simply a colon (":")). We additionally set the entity_type
key for all definitions (as we used in the plugin) and also set the other definition keys (as defined in the annotation) accordingly.
And that's it! If your plugin type implements DerivativeInspectionInterface
(which the normal PluginBase
class does), you also have handy methods for finding out a plugin's base ID and derivative ID (if any). But usually the code using the plugins doesn't need to be aware of derivatives and can simply handle them like any other plugin. Just be aware that this leads to plugin IDs now all potentially containing colons, and not only the usual "alphanumerics plus underscores" ID characters.
A side note about nomenclature
This is a bit confusing actually, especially as older documentation remains unupdated: The new individual plugins that were derived from the base defintion are referred to as "derivative plugin definitions", "plugin derivatives" or just "derivatives". Confusingly, though, the class creating the derivatives was also called a "derivative class" (and the key in the plugin definition was, consequently, derivative
).
In #1875996: Reconsider naming conventions for derivative classes, this discrepancy was discussed and eventually resolved by renaming the classes creating derivative definitions (along with their interfaces, etc.) to "derivers".
If you are reading documentation that is more than a few months old, hopefully this will prevent you from some confusion.
Other posts in this series
- Part 1: Creating an entity type
- Part 2: Configuration and schema
- Part 3: Creating your own service
- Part 4: Creating plugin types
Image credit: DonkeyHotey
Add new comment